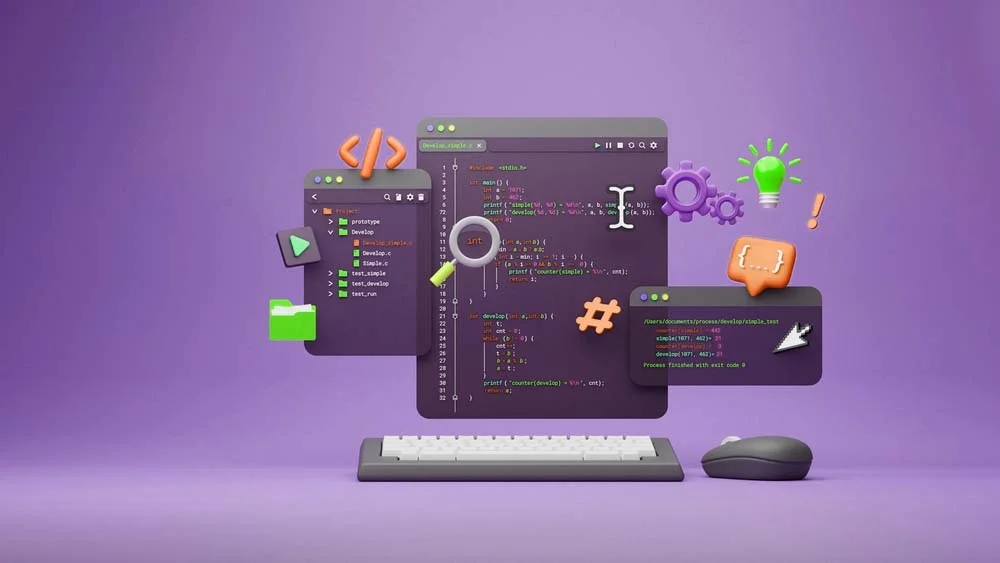
If you’re new to programming, creating tools might seem like a big challenge. But actually, building simple tools is one of the best ways to learn programming and solve real-world problems. Tools can be as simple as scripts that save you time or small programs that automate tasks. In this post, we’ll show you how to get started with creating tools, even if you’re a beginner. We’ll also cover how to think critically while building your tool, how to research technologies you need, and how to automate your tasks.
What is a Tool in Programming?
In programming, a tool is just a program designed to make a task easier or solve a specific problem. A tool could be:
- A script that renames files in a folder
- A program that collects data from websites
- A calculator that helps you perform complex calculations faster
- A tool that automates repetitive tasks, like sending emails
The good news is, you don’t need to be an expert to create useful tools. In fact, building your own tools is a great way to improve your programming skills.
Step 1: Choose a Programming Language
When you’re just starting out, it can be tough to decide which programming language to use. Don’t worry—almost any language can be used to build tools. Here’s a quick guide to some beginner-friendly options:
- Python: Python is perfect for beginners! It has simple, easy-to-read code and is great for automating tasks, processing data, or building small web apps.
- JavaScript: If you want to build web tools or interact with websites, JavaScript is the way to go.
- Ruby: Ruby is another beginner-friendly language, great for building simple tools and web applications.
- Bash/Shell Scripting: If you want to automate tasks on Linux or macOS, bash scripting is very useful and runs directly in your terminal.
- Go (Golang): A good choice if you want to build powerful, fast tools, especially for handling server-side tasks.
Tip: If you’re unsure, start with Python. It’s easy to learn, has tons of tutorials, and is widely used for building tools.
Step 2: Find a Problem to Solve
Now that you’ve chosen a language, the next step is to find a problem you want to solve with a tool. The best way to do this is by thinking of tasks you already do that could be automated or simplified. Here are a few ideas:
- Renaming a group of files in a folder to keep them organized
- Collecting information from a website (like product prices or news articles)
- Sending automated emails or reminders based on a schedule
- Organizing and analyzing data in a CSV or Excel file
Tip: Start small! Pick something that isn’t too complex. It’s better to build a simple tool first and then gradually make it more advanced.
Step 3: Break Down the Problem
Once you’ve picked your problem, it’s time to break it down into smaller, easy-to-understand steps. This is where critical thinking becomes important. Instead of trying to solve everything at once, you’ll focus on solving one small task at a time.
For example, if you want to build a tool that renames all files in a folder to lowercase, here’s how you could break it down:
- Input: Get a list of all files in the folder.
- Processing: Check if each file name is in uppercase.
- Output: Rename each file to be in lowercase.
Breaking the task down helps you focus on one piece at a time, making it easier to code and troubleshoot.
Tip: Before you start coding, ask yourself:
- What do I need to do to solve this problem?
- What are the different steps involved?
- What could go wrong? (e.g., what if there’s no file in the folder?)
Step 4: Start Writing Code
Once you’ve broken down the problem into smaller tasks, you can begin coding. If you’re building a Python script to rename files, here’s a simple version:
import os
def rename_files_in_directory(directory):
for filename in os.listdir(directory): # List all files in the directory
if filename.isupper(): # Check if the filename is in uppercase
new_name = filename.lower() # Convert the filename to lowercase
os.rename(os.path.join(directory, filename), os.path.join(directory, new_name))
# Example usage
rename_files_in_directory('/path/to/directory') # Change to your folder path
This code:
- Uses os.listdir() to get all files in a folder.
- Checks if the file names are uppercase using isupper().
- Renames them using os.rename().
Tip: Write your code in small pieces and test it as you go. You don’t have to make everything perfect right away. The goal is to get a working version first, then improve it.
Step 5: How to Think Critically While Building Your Tool
As you code, it’s important to think critically about your project. This means asking questions, considering different possibilities, and improving your tool step by step. Here’s how:
- Start simple: Write a basic version of your tool that does the main task. Don’t worry about making it perfect.
- Test it often: Check if your tool works with different inputs. For example, test if it works with an empty folder or files with unusual characters.
- Refine your tool: Once your tool works, think about how you can improve it. Could you add a progress bar or an error message if something goes wrong?
Tip: Try to improve your tool by asking:
- How can I make this faster?
- How can I make it more user-friendly?
- What happens if the user inputs something unexpected?
Step 6: Research and Automate Using New Technologies
When building tools, you’ll often need to learn about new technologies, libraries, or methods. This is where research comes in. You might want to add features you don’t know how to code yet. Here’s how to find the information you need:
- Google your problem: Use search engines to look up specific questions like:
- “How to rename files in Python?”
- “How to scrape websites with Python?”
- “How to handle errors in Python?”
- Use online communities: Forums like Stack Overflow, Reddit, or specific programming communities are great for getting help. Search for similar questions or ask for advice.
- Check documentation: Every programming language or library comes with documentation. This is a great resource to learn how specific functions work.
Tip: Don’t be afraid to ask questions online or read through documentation. Most programming problems have already been solved by someone else!
Step 7: Test, Debug, and Refine Your Tool
Testing is an important part of building any tool. After you’ve written your code, you need to test it to make sure it works correctly. Here are some things to consider:
- What happens if there are no files to rename?
- What if the user inputs the wrong folder path?
- What if the tool encounters an error during execution?
Tip: Test your tool with different scenarios to make sure it works in all situations. Fix any bugs or issues that come up.
Step 8: Share, Get Feedback, and Improve
Once your tool works, it’s time to share it with others! Whether it’s your friends, online communities, or just by publishing it on your blog, getting feedback is key. Others might notice things you missed or suggest new features you could add.
Critical Thinking Tip: When you get feedback, think about how you can improve your tool based on that feedback. What could make it even better? Could you make it faster, easier to use, or more powerful?
some suggestions :
- research the topics on stack overflow
- research the topics on different forums
- start learning about the technologies from youtube